10 Must-Know Java Coding Interview Questions
Easy Questions
1. What are the 5 pillars of OOP?
- Encapsulation
- Abstraction
- Inheritance
- Polymorphism
- Reusability
2. What is the difference between a constructor and a method?
- A constructor is a special method that is used to initialize an object.
- A method is a function that is defined inside a class.
3. What is the difference between a static and a non-static method?
- A static method is a method that is defined within a class, but does not require an instance of the class to be called.
- A non-static method is a method that is defined within a class and requires an instance of the class to be called.
Medium Questions
4. What is the difference between an interface and an abstract class?

- An interface is a contract that defines a set of methods that a class must implement.
- An abstract class is a class that defines a set of abstract methods that must be implemented by its subclasses.
5. What is the difference between a final class and a non-final class?

- A final class cannot be extended by other classes.
- A non-final class can be extended by other classes.
6. What is the difference between a private, protected, and public member?
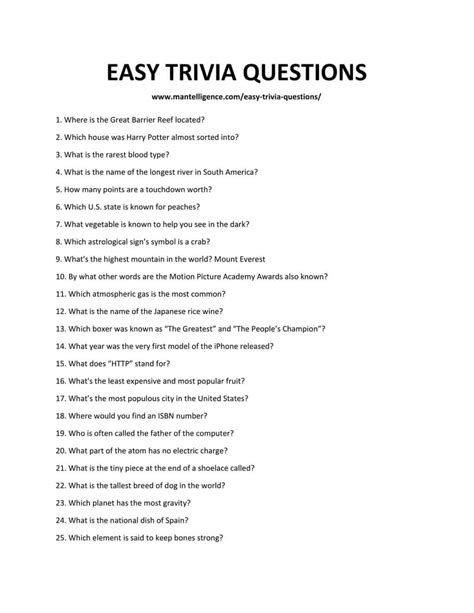
- A private member can only be accessed by the class in which it is defined.
- A protected member can be accessed by the class in which it is defined and its subclasses.
- A public member can be accessed by any class.
Hard Questions
7. What is the difference between a stack and a queue?
- A stack is a data structure that follows the Last In First Out (LIFO) principle, meaning the last item added is the first item removed.
- A queue is a data structure that follows the First In First Out (FIFO) principle, meaning the first item added is the first item removed.
8. What is a binary search tree?
- A binary search tree is a data structure that stores data in a way that allows for efficient searching and retrieval.
- It is implemented using a binary tree, which is a tree in which each node has a maximum of two children.
9. What is a hashtable?

- A hashtable is a data structure that maps keys to values.
- It is implemented using an array of buckets, each of which contains a list of key-value pairs.
10. What is a linked list?
- A linked list is a data structure that stores data in a linear fashion.
- It is implemented using a series of nodes, each of which contains a value and a pointer to the next node.