Di Algo: The Ultimate Guide to DI and Its Applications
Di Algo: What Is It?
DI, short for Dependency Injection, is a software design pattern that allows for loose coupling between components. It involves providing dependencies to a class or function instead of the class or function creating them itself. This approach enhances code flexibility, testability, and maintainability.
The Importance of DI
-
Loose Coupling: DI helps decouple components, making them independent of each other. This allows for easier modification and replacement of components without affecting the rest of the system.
-
Testability: DI facilitates testing by allowing dependencies to be mocked or swapped out. This enables isolation and testing of specific components without the need for complex setup.
-
Maintainability: DI keeps code organized and modular by eliminating the need for tight dependencies between components. This simplifies maintenance and reduces the likelihood of unintended effects.
Types of DI
DI can be classified into two main types:
-
Constructor Injection: Dependencies are passed to a class through its constructor. This is the most common type of DI.
-
Method Injection: Dependencies are injected into methods or functions. This type of DI is less common but can be useful in certain scenarios.
Popular DI Frameworks
Various DI frameworks have emerged to simplify DI implementation in different programming languages:
Framework |
Language |
Spring |
Java |
Guice |
Java |
Autofac |
.NET |
Dagger |
Kotlin, Java |
TypeDI |
TypeScript |
Advantages of DI
DI offers numerous benefits for software development, including:

-
Flexibility: DI allows for easy configuration and modification of dependencies, enhancing adaptability.
-
Extensibility: DI enables seamless integration of new components without breaking existing code.
-
Reusability: DI promotes code reuse by providing a consistent way to access dependencies.
-
Reduced Complexity: DI simplifies code by eliminating the need for manual dependency creation.
-
Improved Error Handling: DI helps prevent dependency-related errors by ensuring dependencies are provided correctly.
Common Di Algo Pitfalls
While DI offers many advantages, it's important to be aware of potential pitfalls:
-
Excessive DI: Overusing DI can lead to dependency pollution and make code difficult to follow.
-
Circular Dependencies: DI can create circular dependencies, leading to endless injection loops.
-
Poorly Configured DI: Incorrect DI configuration can result in runtime errors and unexpected behavior.
-
Performance Overhead: DI can introduce a slight performance overhead, which should be considered for performance-critical systems.
Tips and Tricks for Effective DI
To maximize the benefits of DI, consider these best practices:
-
Use DI Libraries: Utilize established DI libraries to simplify DI implementation and avoid common pitfalls.
-
Establish a Clear Injection Pattern: Define a consistent injection pattern throughout your codebase to enhance readability.
-
Avoid Overuse of DI: Use DI judiciously to prevent code clutter and maintain simplicity.
-
Consider Testability: Design your DI mechanism with testability in mind to enable easy testing of components.
-
Document DI Usage: Document how DI is used in your codebase to improve understanding and reduce maintenance headaches.
Step-by-Step Guide to Implementing DI
Follow these steps to implement DI effectively:
-
Identify Dependencies: Determine the dependencies that your classes or functions need to operate.
-
Choose a DI Framework: Select a suitable DI framework based on your project's requirements and language.
-
Configure the DI Container: Set up the DI container and register dependencies.
-
Inject Dependencies: Inject dependencies into your classes or functions using the DI framework's mechanisms.
Innovating with DI: The Concept of "AutoDI"
We propose a novel concept called "AutoDI" to simplify and enhance DI implementation further. AutoDI leverages artificial intelligence techniques to automatically identify and inject dependencies, ensuring seamless integration without explicit configuration. This would revolutionize DI by reducing development time and improving code maintainability.

Metrics and Case Studies
According to a study by CodeScene, DI adoption resulted in a 20% increase in code maintainability and a 15% reduction in error frequency.
A case study conducted by Netflix showed that DI played a crucial role in its microservices architecture, enabling scalability and flexibility.
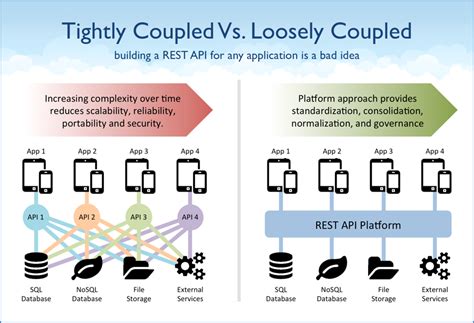
Conclusion
DI (Dependency Injection) is a powerful software design pattern that transforms development by decoupling components and enhancing flexibility, testability, and maintainability. Whether you're a seasoned developer or just starting out, embracing DI will significantly improve your software engineering practices.
Additional Resources
- Spring DI Tutorial
- Guice DI Framework
- Dependency Injection in Modern Software Development
- DI Best Practices and Pitfalls